Summary
- What is CryptPass
- App features
- How it works, in a nutshell
- CryptPass architecture
- App bugs and technical debts
- Contributions
- Downloads and source code
What is CryptPass
CryptPass is a free system for encrypting, storing and managing secret credentials, mainly passwords, within a text file.
This system is composed of two main parts: a library (the core of CryptPass) and an “app” (a graphic interface for the library). I’m aware that many other similar systems have already existed for a long time, and that they are very stable and widely used too. Nonetheless, I wanted to create my own, designed with some peculiarities and small differences compared to similar products, to reflect my philosophy, that can be resumed in the following requirements:
REQUIREMENT | DETAILS |
---|---|
Simplicity | Architecture and functionalities must be simple, since this makes expansion, bugs correction and the entire code handling easier. |
Strength* | The cryptographic functions used and their combination must be quite strong.. |
Focus | The system must perform well only on its specific task: to organize and to encrypt secrets into a string storable anywhere. Any additional feature should be implemented externally. |
Two-ways protection | The cryptographic keys are derived both by a user-created password and by a pseudo-random system-generated token. |
Being local | The system stores data locally (i.e. offline). |
Flexibility | Users should not have any additional constraints than what the system requires for security reasons. |
Openness | The source code must be open. |
Being free | The system must be free software-licensed. |
Reusability | The system must be primarily a reusable library, ready for any contexts. |
Being cross-platform* | The system must be cross-platform. |
* As of now, these aspects are not completely satisfied: Strength, because the system is too young and was not sufficiently analyzed or tested by community yet; being cross-platform, because only the library, compatible with node.js or pure javascript, is really cross-platform while the “app”, for the moment, only supports Android, Linux and Windows-operating systems.
App features
At the moment, CryptPass has the following features:
FEATURE | DETAILS |
---|---|
Multi-OS Support | CryptPass runs on Android, Linux and Windows (see downloads) |
Two-ways protection | CryptPass protects your secrets in two ways at the same time:
|
Description | You can set a description of the wallet (that won’t be encrypted) |
Secrets storage | You can store your secrets in a collection of entries, each of which has:
|
Secrets search engine | By default, entries are listed in alphabetical order. When you store a huge amount of entries, they can also be retrieved more comfortably in one of the following ways:
|
Managing | You can also: view the secret sequence, change the master password and restore or reset the wallet |
How it works, in a nutshell
CryptPass App simply and safely stores your secret credentials into a file, in a location of your filesystem of your own choosing. In fact, your credentials are completely encrypted with a password that you create (master password) before saving them into the file. To read your credentials, you just have to type your master password.
If you want your secrets to be safely stored (for example in case your device stops working or has to be reset), you can use your favorite cloud data storage service (like Dropbox and many others) to keep an always updated replica of your CryptPass file. But remember, only in case of restoring that file, along with your master password, you must also type the sequence number CryptPass provided during the initiation and viewable at any time using the specific app menu item (other options → view sequence).
CryptPass architecture
As said above, CryptPass is primarily a library, written in Typescript and using NodeJS libraries. CryptPassApp is an application whose aims are: to provide a graphical interface to CryptPass library, to implement the read/write functions for storing encrypted secrets and to be cross-platform. More technical and architectural details as follows.
The library
The core of CryptPass is the library. This library is NodeJS native (with full performance) but, in order for it to be also used in pure javascript, even if with some performance loss, some specific bundling libraries were added ("browser-resolve", "buffer", "crypto-browserify", "randombytes-pure", "scrypt-js", "stream-browserify").
The library completely abstracts the mass storage layer of the two main data structures, which are: KeyPass (containing the encrypted secrets) and Sequence (containing the secret sequence number). In fact, to instantiate the library, it’s necessary to pass as input the following 4 parameters: 1) the KeyPass (i.e. the encrypted secrets data structure); 2) the Sequence (i.e. the secret sequence number); 3) the KeyPassWriter and 4) the SequenceWriter, both functions that take as input argument the content of the respective data structures and provide a storage mechanism (for example they could write data as a file on filesystem).
We can distinguish two main components of the library: cryptographic APIs and entries management APIs. Since the latter is just a database-like collection of methods that simply add/edit/delete entries in a JSON data structure (implicitly described by the previous section “app feature” within the “secrets storage” item), we’ll only focus on the cryptographic APIs.
The cryptographic scheme is the following:
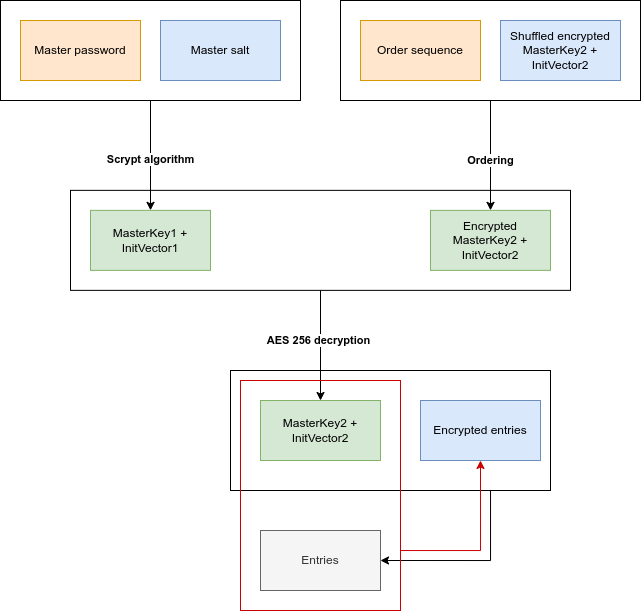
In the above diagram, in orange we have the elements known only by the user: the master password and the numeric sequence (order sequence). In blue we have the elements stored together inside the same data structure (named KeyPass inside the source code). Finally, in green, we have the elements derived from the cryptographic operation between these elements and in gray we have the cleartext entries (stored in RAM) that could be encrypted. Either for encryption and decryption purposes, the first two levels of the diagram are executed because they are necessary to calculate the couple called “MasterKey2 + InitVector2” with which the library encrypts and decrypts the wallet entries (in this case with the algorithm aes-256).
Most of that cryptographic sequence is quite common in this kind of softwares: a couple “key / initial vector” is derived from a password with a strong hash function (scrypt in this case) and is used to encrypt/decrypt some cleartext/ciphertext. On the other hand, it is worth explaining the only uncommon part: the ordering sequence. Let’s start with the aim. If any criminal obtained your KeyPass data structure and, with social engineering techniques, discovered your master password, he could easily decrypt all your credentials. But it wouldn’t need to be a criminal. Suppose the KeyPass data structure is stored in a cloud storage service and the user uses the same master password both for the cloud service and for the KeyPass; in this case, the cloud storage service could potentially use that password to decrypt the whole KeyPass data. So the “sequence number” is useful to mitigate this vulnerability. First, CryptPass generates a pseudo-random sequence of numbers (from 0 to 25), then it chunks the master key into 26 segments ordered by the sequence and that shuffled master key is stored inside KeyPass data structure. Only the user knows the exact order (i.e. sequence number) that correctly rebuilds the master key, and, thanks to this, he’s even more protected (brute forcing a sequence of 26 numbers is combinatorially hard).
To easily apply the “sequence number” trick and to easily provide a master password changing operation, a secondary master key was introduced. So first the primary master key is derived by scrypt from the concatenation of user master password and master salt. Then the encrypted secondary master key chunks are reordered. That done, the secondary master key is decrypted by the primary master key and is used in turn to encrypt / decrypt wallet entries.
The app
CryptPass app is written in Typescript with Apache Cordova and optimized for Android (with Cordova native platform support), Linux and Windows (both with Cordova Electron platform support). The app user interface and functionalities are exactly the same across all the supported operating systems except for storage management, as recaped as follows:
STORAGE FEATURE | ANDROID | LINUX / WINDOWS |
---|---|---|
KeyPass file initialization | The KeyPass file is created by the app after selecting the desired location | The KeyPass file must already exist (as an empty text file) and then must be selected from the app |
Sequence number storage | The sequence number is encrypted within the Android Keystore system | The sequence number is not encrypted (but it’s not easy to steal) |
The app uses the CryptPass library in bundled version (i.e. browser compatible) and enriches it with the graphics and all the due managing and configuration features to be effectively user interactive. The graphic (actually an embedded website) is quite essential and it uses the basic Bootstrap features. The backend parts of the code also have a specific layer to abstract the different storage managing across the different platforms.
The Android version also uses a customized version of two plugins for reading and writing files on device filesystem. Original plugins, in fact, didn’t permit all the operations needed, especially for granting permanent permission on files to be read and written, also after the device has been rebooted. The source code of both customizations can be found here:
Here’s a screenshot of the starting view:
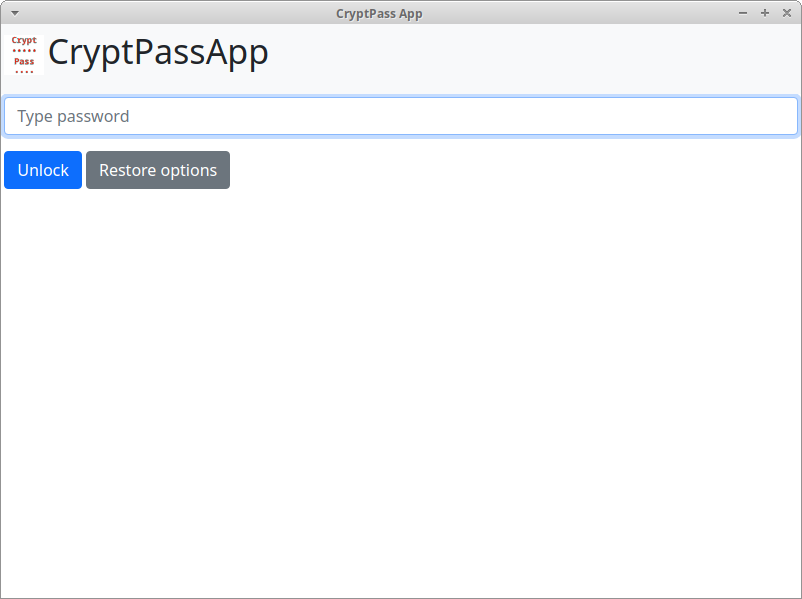
App bugs and technical debts
For the moment, CryptPass app is sufficiently usable but with several compromises (or sometimes actual bugs) that anybody can help me solve (and thanks in advance!). The only ones known to me are summarized as follows, and none of these have to do with vulnerability or security weakness:
Title | Category | Platform | Description |
---|---|---|---|
Uncomplete cross-platform | Technical debt | ALL | Since I have only PCs with linux inside and only a smartphone with Android, I could code the app for a restricted number of OS. Cordova permits to extend the support for iOS and MacOS, and also to have a native installer for Windows (now only a zipped portable version is provided for it). |
File creation on Electron | Technical debt | Linux and Windows | I have not implemented the native Electron showOpenDialog() method so, for the first usage, the password file has to be created manually by the user as an empty text file |
Windows antivirus | BUG | Windows | I tried CryptPass only once on a Windows desktop PC and it seems that Avast antivirus considered it as malicious because of the capabilities to read and write files (even if it’s just the password file). I haven’t explored any solutions yet but, as a workaround, adding an exception for the CryptPass installation folder (https://support.avast.com/en-ww/article/Antivirus-scan-exclusions). |
Android permissions | BUG | Android | If a cloud storage synchronization service updates the KeyPass file, CryptPass loses reading (and writing?) permissions on that file. To investigate the causes, please look at the customized plugins I used:
|
Multi-wallet support | Technical debt | ALL | At the moment, the app can manage one wallet per time (represented by the couple “KeyPass file” and “sequence number”) |
Languages support | Technical debt | ALL | At the moment, only my (mediocre) english is supported. Please correct me about any errors or typos and feel free to suggest any better linguistic formulations. Also the app was not natively set up for multi-language support but, with a few edits, it could be. |
Contributions
This is free software and any contribution is welcome!
You can collaborate with me on the source code (look at the next chapter for links) and you can also send me any issue always through github or emailing me (look at the bottom of this page to find my address).
Issues links
For CryptPass library https://github.com/ossacolsale/CryptPass/issues
For CryprPass app https://github.com/ossacolsale/CryptPassApp/issues
Downloads and source code
Install on Android
CryptPass app is on Google Play Store: https://play.google.com/store/apps/details?id=com.cryptpass.app
Install on Linux
Here the builded versions for Linux:
Ubuntu / Ubuntu-derived / Debian (.deb package)
Install on Windows
Source code
For each supported platforms, you can also manually build the CryptPass library and CryptPass app downloading the source code from Github:
_